Javascript
Time Zone Clock
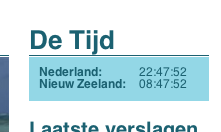
Recently I created a site for a family who were travelling around the world. They asked me if I could create 2 time clocks that would show the time in there present location and the time of there home country (the Netherlands).
It was one of my first
encounters with the Date
object of Javascript.
Perhabs by writing this tutorial/ article I will understand
it some more for future use.
This article is not yet finished.
The Script
First I will show you the complete script. After that I will describe what it does.
function clock() { var hourOffset = getTimeOffset(); var locOffset = hourOffset * 60 * 60 * 1000; var NLOffset = 2 * 60 * 60 * 1000; var oLocal = new Date(); var off = ( oLocal.getTimezoneOffset() ) * 60 * 1000; var timeStamp = oLocal.getTime() + off; var oGMT = new Date(); oGMT.setTime( timeStamp ); var oNLLoc = new Date(); var NLTimeStamp = oGMT.getTime() + NLOffset; oNLLoc.setTime( NLTimeStamp ); var sDateTimeL1 = leadingZero(oNLLoc.getHours()) + ":" + leadingZero(oNLLoc.getMinutes()) + ":" + leadingZero(oNLLoc.getSeconds()); elementInnerHTML('firstloc', sDateTimeL1 ); var sDateTimeL2 = leadingZero(oGMT.getHours()) + ":" + leadingZero(oGMT.getMinutes()) + ":" + leadingZero(oGMT.getSeconds()); var oLoc = new Date(); var newLocTimeStamp = oGMT.getTime() + locOffset; oLoc.setTime( newLocTimeStamp ); var sDateTimeL3 = leadingZero(oLoc.getHours()) + ":" + leadingZero(oLoc.getMinutes()) + ":" + leadingZero(oLoc.getSeconds()); elementInnerHTML('secondloc', sDateTimeL3 ); setTimeout("clock()", 1000); } function elementInnerHTML( id , text ) { if (document.getElementById) { x = document.getElementById(id); if ( x == null ) { return; } x.innerHTML = ''; x.innerHTML = text; } else if (document.all) { x = document.all[id]; if ( x == null ) { return; } x.innerHTML = text; } else if (document.layers) { x = document.layers[id]; if ( x == null ) { return; } text2 = '<P CLASS="testclass">' + text + '</P>'; x.document.open(); x.document.write(text2); x.document.close(); } } function takeYear(theDate) { x = theDate.getYear(); var y = x % 100; y += (y < 38) ? 2000 : 1900; return y; } function leadingZero(nr) { if (nr < 10) nr = "0" + nr; return nr; } function getTimeOffset() { if (document.getElementById) { x = document.getElementById('timeoffset'); } else if (document.all) { x = document.all['timeoffset']; } if ( x == null ) { return; } if( x.style.display != 'none') { x.style.display = 'none'; } return x.childNodes[0].nodeValue; }